forked from javadev/LeetCode-in-Kotlin
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
12 changed files
with
478 additions
and
0 deletions.
There are no files selected for viewing
27 changes: 27 additions & 0 deletions
27
.../g3101_3200/s3105_longest_strictly_increasing_or_strictly_decreasing_subarray/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
package g3101_3200.s3105_longest_strictly_increasing_or_strictly_decreasing_subarray | ||
|
||
// #Easy #Array #2024_04_13_Time_159_ms_(94.00%)_Space_36.4_MB_(92.00%) | ||
|
||
import kotlin.math.max | ||
|
||
class Solution { | ||
fun longestMonotonicSubarray(nums: IntArray): Int { | ||
var inc = 1 | ||
var dec = 1 | ||
var res = 1 | ||
for (i in 1 until nums.size) { | ||
if (nums[i] > nums[i - 1]) { | ||
inc += 1 | ||
dec = 1 | ||
} else if (nums[i] < nums[i - 1]) { | ||
dec += 1 | ||
inc = 1 | ||
} else { | ||
inc = 1 | ||
dec = 1 | ||
} | ||
res = max(res, max(inc, dec)) | ||
} | ||
return res | ||
} | ||
} |
52 changes: 52 additions & 0 deletions
52
...200/s3105_longest_strictly_increasing_or_strictly_decreasing_subarray/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,52 @@ | ||
3105\. Longest Strictly Increasing or Strictly Decreasing Subarray | ||
|
||
Easy | ||
|
||
You are given an array of integers `nums`. Return _the length of the **longest** subarray of_ `nums` _which is either **strictly increasing** or **strictly decreasing**_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,4,3,3,2] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** | ||
|
||
The strictly increasing subarrays of `nums` are `[1]`, `[2]`, `[3]`, `[3]`, `[4]`, and `[1,4]`. | ||
|
||
The strictly decreasing subarrays of `nums` are `[1]`, `[2]`, `[3]`, `[3]`, `[4]`, `[3,2]`, and `[4,3]`. | ||
|
||
Hence, we return `2`. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [3,3,3,3] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** | ||
|
||
The strictly increasing subarrays of `nums` are `[3]`, `[3]`, `[3]`, and `[3]`. | ||
|
||
The strictly decreasing subarrays of `nums` are `[3]`, `[3]`, `[3]`, and `[3]`. | ||
|
||
Hence, we return `1`. | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [3,2,1] | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** | ||
|
||
The strictly increasing subarrays of `nums` are `[3]`, `[2]`, and `[1]`. | ||
|
||
The strictly decreasing subarrays of `nums` are `[3]`, `[2]`, `[1]`, `[3,2]`, `[2,1]`, and `[3,2,1]`. | ||
|
||
Hence, we return `3`. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= nums.length <= 50` | ||
* `1 <= nums[i] <= 50` |
30 changes: 30 additions & 0 deletions
30
...3200/s3106_lexicographically_smallest_string_after_operations_with_constraint/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
package g3101_3200.s3106_lexicographically_smallest_string_after_operations_with_constraint | ||
|
||
// #Medium #String #Greedy #2024_04_13_Time_162_ms_(74.19%)_Space_36.2_MB_(77.42%) | ||
|
||
import kotlin.math.abs | ||
import kotlin.math.min | ||
|
||
@Suppress("NAME_SHADOWING") | ||
class Solution { | ||
fun getSmallestString(s: String, k: Int): String { | ||
var k = k | ||
val sArray = s.toCharArray() | ||
for (i in sArray.indices) { | ||
val distToA = cyclicDistance(sArray[i], 'a') | ||
if (distToA <= k) { | ||
sArray[i] = 'a' | ||
k -= distToA | ||
} else if (k > 0) { | ||
sArray[i] = (sArray[i].code - k).toChar() | ||
k = 0 | ||
} | ||
} | ||
return String(sArray) | ||
} | ||
|
||
private fun cyclicDistance(ch1: Char, ch2: Char): Int { | ||
val dist = abs(ch1.code - ch2.code) | ||
return min(dist, (26 - dist)) | ||
} | ||
} |
51 changes: 51 additions & 0 deletions
51
...06_lexicographically_smallest_string_after_operations_with_constraint/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
3106\. Lexicographically Smallest String After Operations With Constraint | ||
|
||
Medium | ||
|
||
You are given a string `s` and an integer `k`. | ||
|
||
Define a function <code>distance(s<sub>1</sub>, s<sub>2</sub>)</code> between two strings <code>s<sub>1</sub></code> and <code>s<sub>2</sub></code> of the same length `n` as: | ||
|
||
* The **sum** of the **minimum distance** between <code>s<sub>1</sub>[i]</code> and <code>s<sub>2</sub>[i]</code> when the characters from `'a'` to `'z'` are placed in a **cyclic** order, for all `i` in the range `[0, n - 1]`. | ||
|
||
For example, `distance("ab", "cd") == 4`, and `distance("a", "z") == 1`. | ||
|
||
You can **change** any letter of `s` to **any** other lowercase English letter, **any** number of times. | ||
|
||
Return a string denoting the **lexicographically smallest** string `t` you can get after some changes, such that `distance(s, t) <= k`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "zbbz", k = 3 | ||
|
||
**Output:** "aaaz" | ||
|
||
**Explanation:** | ||
|
||
Change `s` to `"aaaz"`. The distance between `"zbbz"` and `"aaaz"` is equal to `k = 3`. | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "xaxcd", k = 4 | ||
|
||
**Output:** "aawcd" | ||
|
||
**Explanation:** | ||
|
||
The distance between "xaxcd" and "aawcd" is equal to k = 4. | ||
|
||
**Example 3:** | ||
|
||
**Input:** s = "lol", k = 0 | ||
|
||
**Output:** "lol" | ||
|
||
**Explanation:** | ||
|
||
It's impossible to change any character as `k = 0`. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= s.length <= 100` | ||
* `0 <= k <= 2000` | ||
* `s` consists only of lowercase English letters. |
32 changes: 32 additions & 0 deletions
32
...kotlin/g3101_3200/s3107_minimum_operations_to_make_median_of_array_equal_to_k/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
package g3101_3200.s3107_minimum_operations_to_make_median_of_array_equal_to_k | ||
|
||
// #Medium #Array #Sorting #Greedy #2024_04_13_Time_554_ms_(100.00%)_Space_82.2_MB_(68.00%) | ||
|
||
import kotlin.math.abs | ||
|
||
class Solution { | ||
fun minOperationsToMakeMedianK(nums: IntArray, k: Int): Long { | ||
nums.sort() | ||
val n = nums.size | ||
val medianIndex = n / 2 | ||
var result: Long = 0 | ||
var totalElements = 0 | ||
var totalSum: Long = 0 | ||
var i = medianIndex | ||
if (nums[medianIndex] > k) { | ||
while (i >= 0 && nums[i] > k) { | ||
totalElements += 1 | ||
totalSum += nums[i].toLong() | ||
i -= 1 | ||
} | ||
} else if (nums[medianIndex] < k) { | ||
while (i < n && nums[i] < k) { | ||
totalElements += 1 | ||
totalSum += nums[i].toLong() | ||
i += 1 | ||
} | ||
} | ||
result += abs(totalSum - (totalElements.toLong() * k)) | ||
return result | ||
} | ||
} |
45 changes: 45 additions & 0 deletions
45
...3101_3200/s3107_minimum_operations_to_make_median_of_array_equal_to_k/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
3107\. Minimum Operations to Make Median of Array Equal to K | ||
|
||
Medium | ||
|
||
You are given an integer array `nums` and a **non-negative** integer `k`. In one operation, you can increase or decrease any element by 1. | ||
|
||
Return the **minimum** number of operations needed to make the **median** of `nums` _equal_ to `k`. | ||
|
||
The median of an array is defined as the middle element of the array when it is sorted in non-decreasing order. If there are two choices for a median, the larger of the two values is taken. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [2,5,6,8,5], k = 4 | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** | ||
|
||
We can subtract one from `nums[1]` and `nums[4]` to obtain `[2, 4, 6, 8, 4]`. The median of the resulting array is equal to `k`. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [2,5,6,8,5], k = 7 | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** | ||
|
||
We can add one to `nums[1]` twice and add one to `nums[2]` once to obtain `[2, 7, 7, 8, 5]`. | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [1,2,3,4,5,6], k = 4 | ||
|
||
**Output:** 0 | ||
|
||
**Explanation:** | ||
|
||
The median of the array is already equal to `k`. | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= nums.length <= 2 * 10<sup>5</sup></code> | ||
* <code>1 <= nums[i] <= 10<sup>9</sup></code> | ||
* <code>1 <= k <= 10<sup>9</sup></code> |
81 changes: 81 additions & 0 deletions
81
src/main/kotlin/g3101_3200/s3108_minimum_cost_walk_in_weighted_graph/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,81 @@ | ||
package g3101_3200.s3108_minimum_cost_walk_in_weighted_graph | ||
|
||
// #Hard #Array #Bit_Manipulation #Graph #Union_Find | ||
// #2024_04_13_Time_791_ms_(100.00%)_Space_139.3_MB_(26.67%) | ||
|
||
@Suppress("NAME_SHADOWING") | ||
class Solution { | ||
fun minimumCost(n: Int, edges: Array<IntArray>, query: Array<IntArray>): IntArray { | ||
val parent = IntArray(n) | ||
val bitwise = IntArray(n) | ||
val size = IntArray(n) | ||
var i = 0 | ||
while (i < n) { | ||
parent[i] = i | ||
size[i] = 1 | ||
bitwise[i] = -1 | ||
i++ | ||
} | ||
val len = edges.size | ||
i = 0 | ||
while (i < len) { | ||
val node1 = edges[i][0] | ||
val node2 = edges[i][1] | ||
val weight = edges[i][2] | ||
val parent1 = findParent(node1, parent) | ||
val parent2 = findParent(node2, parent) | ||
if (parent1 == parent2) { | ||
bitwise[parent1] = bitwise[parent1] and weight | ||
} else { | ||
var bitwiseVal: Int | ||
val check1 = bitwise[parent1] == -1 | ||
val check2 = bitwise[parent2] == -1 | ||
bitwiseVal = if (check1 && check2) { | ||
weight | ||
} else if (check1) { | ||
weight and bitwise[parent2] | ||
} else if (check2) { | ||
weight and bitwise[parent1] | ||
} else { | ||
weight and bitwise[parent1] and bitwise[parent2] | ||
} | ||
if (size[parent1] >= size[parent2]) { | ||
parent[parent2] = parent1 | ||
size[parent1] += size[parent2] | ||
bitwise[parent1] = bitwiseVal | ||
} else { | ||
parent[parent1] = parent2 | ||
size[parent2] += size[parent1] | ||
bitwise[parent2] = bitwiseVal | ||
} | ||
} | ||
i++ | ||
} | ||
val queryLen = query.size | ||
val result = IntArray(queryLen) | ||
i = 0 | ||
while (i < queryLen) { | ||
val start = query[i][0] | ||
val end = query[i][1] | ||
val parentStart = findParent(start, parent) | ||
val parentEnd = findParent(end, parent) | ||
if (start == end) { | ||
result[i] = 0 | ||
} else if (parentStart == parentEnd) { | ||
result[i] = bitwise[parentStart] | ||
} else { | ||
result[i] = -1 | ||
} | ||
i++ | ||
} | ||
return result | ||
} | ||
|
||
private fun findParent(node: Int, parent: IntArray): Int { | ||
var node = node | ||
while (parent[node] != node) { | ||
node = parent[node] | ||
} | ||
return node | ||
} | ||
} |
54 changes: 54 additions & 0 deletions
54
src/main/kotlin/g3101_3200/s3108_minimum_cost_walk_in_weighted_graph/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,54 @@ | ||
3108\. Minimum Cost Walk in Weighted Graph | ||
|
||
Hard | ||
|
||
There is an undirected weighted graph with `n` vertices labeled from `0` to `n - 1`. | ||
|
||
You are given the integer `n` and an array `edges`, where <code>edges[i] = [u<sub>i</sub>, v<sub>i</sub>, w<sub>i</sub>]</code> indicates that there is an edge between vertices <code>u<sub>i</sub></code> and <code>v<sub>i</sub></code> with a weight of <code>w<sub>i</sub></code>. | ||
|
||
A walk on a graph is a sequence of vertices and edges. The walk starts and ends with a vertex, and each edge connects the vertex that comes before it and the vertex that comes after it. It's important to note that a walk may visit the same edge or vertex more than once. | ||
|
||
The **cost** of a walk starting at node `u` and ending at node `v` is defined as the bitwise `AND` of the weights of the edges traversed during the walk. In other words, if the sequence of edge weights encountered during the walk is <code>w<sub>0</sub>, w<sub>1</sub>, w<sub>2</sub>, ..., w<sub>k</sub></code>, then the cost is calculated as <code>w<sub>0</sub> & w<sub>1</sub> & w<sub>2</sub> & ... & w<sub>k</sub></code>, where `&` denotes the bitwise `AND` operator. | ||
|
||
You are also given a 2D array `query`, where <code>query[i] = [s<sub>i</sub>, t<sub>i</sub>]</code>. For each query, you need to find the minimum cost of the walk starting at vertex <code>s<sub>i</sub></code> and ending at vertex <code>t<sub>i</sub></code>. If there exists no such walk, the answer is `-1`. | ||
|
||
Return _the array_ `answer`_, where_ `answer[i]` _denotes the **minimum** cost of a walk for query_ `i`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** n = 5, edges = [[0,1,7],[1,3,7],[1,2,1]], query = [[0,3],[3,4]] | ||
|
||
**Output:** [1,-1] | ||
|
||
**Explanation:** | ||
|
||
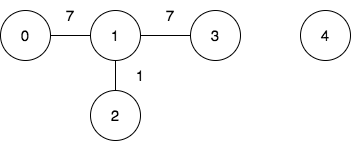 | ||
|
||
To achieve the cost of 1 in the first query, we need to move on the following edges: `0->1` (weight 7), `1->2` (weight 1), `2->1` (weight 1), `1->3` (weight 7). | ||
|
||
In the second query, there is no walk between nodes 3 and 4, so the answer is -1. | ||
|
||
**Example 2:** | ||
|
||
**Input:** n = 3, edges = [[0,2,7],[0,1,15],[1,2,6],[1,2,1]], query = [[1,2]] | ||
|
||
**Output:** [0] | ||
|
||
**Explanation:** | ||
|
||
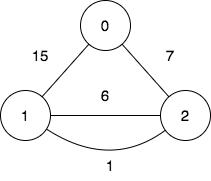 | ||
|
||
To achieve the cost of 0 in the first query, we need to move on the following edges: `1->2` (weight 1), `2->1` (weight 6), `1->2` (weight 1). | ||
|
||
**Constraints:** | ||
|
||
* <code>2 <= n <= 10<sup>5</sup></code> | ||
* <code>0 <= edges.length <= 10<sup>5</sup></code> | ||
* `edges[i].length == 3` | ||
* <code>0 <= u<sub>i</sub>, v<sub>i</sub> <= n - 1</code> | ||
* <code>u<sub>i</sub> != v<sub>i</sub></code> | ||
* <code>0 <= w<sub>i</sub> <= 10<sup>5</sup></code> | ||
* <code>1 <= query.length <= 10<sup>5</sup></code> | ||
* `query[i].length == 2` | ||
* <code>0 <= s<sub>i</sub>, t<sub>i</sub> <= n - 1</code> | ||
* <code>s<sub>i</sub> != t<sub>i</sub></code> |
Oops, something went wrong.