forked from javadev/LeetCode-in-Kotlin
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
18 changed files
with
805 additions
and
0 deletions.
There are no files selected for viewing
21 changes: 21 additions & 0 deletions
21
...in/kotlin/g3001_3100/s3038_maximum_number_of_operations_with_the_same_score_i/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
package g3001_3100.s3038_maximum_number_of_operations_with_the_same_score_i | ||
|
||
// #Easy #Array #Simulation #2024_03_06_Time_142_ms_(100.00%)_Space_34.9_MB_(57.78%) | ||
|
||
class Solution { | ||
fun maxOperations(nums: IntArray): Int { | ||
var c = 1 | ||
var i = 2 | ||
val s = nums[0] + nums[1] | ||
val l = nums.size - (if (nums.size % 2 == 0) 0 else 1) | ||
while (i < l) { | ||
if (nums[i] + nums[i + 1] == s) { | ||
c++ | ||
} else { | ||
break | ||
} | ||
i += 2 | ||
} | ||
return c | ||
} | ||
} |
41 changes: 41 additions & 0 deletions
41
...n/g3001_3100/s3038_maximum_number_of_operations_with_the_same_score_i/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
3038\. Maximum Number of Operations With the Same Score I | ||
|
||
Easy | ||
|
||
Given an array of integers called `nums`, you can perform the following operation while `nums` contains **at least** `2` elements: | ||
|
||
* Choose the first two elements of `nums` and delete them. | ||
|
||
The **score** of the operation is the sum of the deleted elements. | ||
|
||
Your task is to find the **maximum** number of operations that can be performed, such that **all operations have the same score**. | ||
|
||
Return _the **maximum** number of operations possible that satisfy the condition mentioned above_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [3,2,1,4,5] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** We perform the following operations: | ||
- Delete the first two elements, with score 3 + 2 = 5, nums = [1,4,5]. | ||
- Delete the first two elements, with score 1 + 4 = 5, nums = [5]. | ||
|
||
We are unable to perform any more operations as nums contain only 1 element. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [3,2,6,1,4] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** We perform the following operations: | ||
- Delete the first two elements, with score 3 + 2 = 5, nums = [6,1,4]. | ||
|
||
We are unable to perform any more operations as the score of the next operation isn't the same as the previous one. | ||
|
||
**Constraints:** | ||
|
||
* `2 <= nums.length <= 100` | ||
* `1 <= nums[i] <= 1000` |
27 changes: 27 additions & 0 deletions
27
src/main/kotlin/g3001_3100/s3039_apply_operations_to_make_string_empty/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
package g3001_3100.s3039_apply_operations_to_make_string_empty | ||
|
||
// #Medium #Array #Hash_Table #Sorting #Counting | ||
// #2024_03_06_Time_335_ms_(97.73%)_Space_49.8_MB_(81.82%) | ||
|
||
import kotlin.math.max | ||
|
||
class Solution { | ||
fun lastNonEmptyString(s: String): String { | ||
val freq = IntArray(26) | ||
val ar = s.toCharArray() | ||
val n = ar.size | ||
var max = 1 | ||
val sb = StringBuilder() | ||
for (c in ar) { | ||
freq[c.code - 'a'.code]++ | ||
max = max(freq[c.code - 'a'.code].toDouble(), max.toDouble()).toInt() | ||
} | ||
for (i in n - 1 downTo 0) { | ||
if (freq[ar[i].code - 'a'.code] == max) { | ||
sb.append(ar[i]) | ||
freq[ar[i].code - 'a'.code] = 0 | ||
} | ||
} | ||
return sb.reverse().toString() | ||
} | ||
} |
41 changes: 41 additions & 0 deletions
41
src/main/kotlin/g3001_3100/s3039_apply_operations_to_make_string_empty/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
3039\. Apply Operations to Make String Empty | ||
|
||
Medium | ||
|
||
You are given a string `s`. | ||
|
||
Consider performing the following operation until `s` becomes **empty**: | ||
|
||
* For **every** alphabet character from `'a'` to `'z'`, remove the **first** occurrence of that character in `s` (if it exists). | ||
|
||
For example, let initially `s = "aabcbbca"`. We do the following operations: | ||
|
||
* Remove the underlined characters <code>s = "<ins>**a**</ins>a**<ins>bc</ins>**bbca"</code>. The resulting string is `s = "abbca"`. | ||
* Remove the underlined characters <code>s = "<ins>**ab**</ins>b<ins>**c**</ins>a"</code>. The resulting string is `s = "ba"`. | ||
* Remove the underlined characters <code>s = "<ins>**ba**</ins>"</code>. The resulting string is `s = ""`. | ||
|
||
Return _the value of the string_ `s` _right **before** applying the **last** operation_. In the example above, answer is `"ba"`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "aabcbbca" | ||
|
||
**Output:** "ba" | ||
|
||
**Explanation:** Explained in the statement. | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "abcd" | ||
|
||
**Output:** "abcd" | ||
|
||
**Explanation:** We do the following operation: | ||
- Remove the underlined characters s = "<ins>**abcd**</ins>". The resulting string is s = "". | ||
|
||
The string just before the last operation is "abcd". | ||
|
||
**Constraints:** | ||
|
||
* <code>1 <= s.length <= 5 * 10<sup>5</sup></code> | ||
* `s` consists only of lowercase English letters. |
71 changes: 71 additions & 0 deletions
71
...n/kotlin/g3001_3100/s3040_maximum_number_of_operations_with_the_same_score_ii/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,71 @@ | ||
package g3001_3100.s3040_maximum_number_of_operations_with_the_same_score_ii | ||
|
||
// #Medium #Array #Dynamic_Programming #Memoization | ||
// #2024_03_06_Time_179_ms_(100.00%)_Space_38.4_MB_(100.00%) | ||
|
||
import java.util.Objects | ||
import kotlin.math.max | ||
|
||
class Solution { | ||
private lateinit var nums: IntArray | ||
|
||
private var maxOps = 1 | ||
|
||
private val dp: MutableMap<Pos, Int> = HashMap() | ||
|
||
private class Pos(var start: Int, var end: Int, var sum: Int) { | ||
override fun equals(other: Any?): Boolean { | ||
if (other == null) { | ||
return false | ||
} | ||
if (other !is Pos) { | ||
return false | ||
} | ||
return start == other.start && end == other.end && sum == other.sum | ||
} | ||
|
||
override fun hashCode(): Int { | ||
return Objects.hash(start, end, sum) | ||
} | ||
} | ||
|
||
fun maxOperations(nums: IntArray): Int { | ||
this.nums = nums | ||
val length = nums.size | ||
|
||
maxOperations(2, length - 1, nums[0] + nums[1], 1) | ||
maxOperations(0, length - 3, nums[length - 2] + nums[length - 1], 1) | ||
maxOperations(1, length - 2, nums[0] + nums[length - 1], 1) | ||
|
||
return maxOps | ||
} | ||
|
||
private fun maxOperations(start: Int, end: Int, sum: Int, nOps: Int) { | ||
if (start >= end) { | ||
return | ||
} | ||
|
||
if ((((end - start) / 2) + nOps) < maxOps) { | ||
return | ||
} | ||
|
||
val pos = Pos(start, end, sum) | ||
val posNops = dp[pos] | ||
if (posNops != null && posNops >= nOps) { | ||
return | ||
} | ||
dp[pos] = nOps | ||
if (nums[start] + nums[start + 1] == sum) { | ||
maxOps = max(maxOps, (nOps + 1)) | ||
maxOperations(start + 2, end, sum, nOps + 1) | ||
} | ||
if (nums[end - 1] + nums[end] == sum) { | ||
maxOps = max(maxOps, (nOps + 1)) | ||
maxOperations(start, end - 2, sum, nOps + 1) | ||
} | ||
if (nums[start] + nums[end] == sum) { | ||
maxOps = max(maxOps, (nOps + 1)) | ||
maxOperations(start + 1, end - 1, sum, nOps + 1) | ||
} | ||
} | ||
} |
45 changes: 45 additions & 0 deletions
45
.../g3001_3100/s3040_maximum_number_of_operations_with_the_same_score_ii/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
3040\. Maximum Number of Operations With the Same Score II | ||
|
||
Medium | ||
|
||
Given an array of integers called `nums`, you can perform **any** of the following operation while `nums` contains **at least** `2` elements: | ||
|
||
* Choose the first two elements of `nums` and delete them. | ||
* Choose the last two elements of `nums` and delete them. | ||
* Choose the first and the last elements of `nums` and delete them. | ||
|
||
The **score** of the operation is the sum of the deleted elements. | ||
|
||
Your task is to find the **maximum** number of operations that can be performed, such that **all operations have the same score**. | ||
|
||
Return _the **maximum** number of operations possible that satisfy the condition mentioned above_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [3,2,1,2,3,4] | ||
|
||
**Output:** 3 | ||
|
||
**Explanation:** We perform the following operations: | ||
- Delete the first two elements, with score 3 + 2 = 5, nums = [1,2,3,4]. | ||
- Delete the first and the last elements, with score 1 + 4 = 5, nums = [2,3]. | ||
- Delete the first and the last elements, with score 2 + 3 = 5, nums = []. | ||
|
||
We are unable to perform any more operations as nums is empty. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [3,2,6,1,4] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** We perform the following operations: | ||
- Delete the first two elements, with score 3 + 2 = 5, nums = [6,1,4]. | ||
- Delete the last two elements, with score 1 + 4 = 5, nums = [6]. | ||
|
||
It can be proven that we can perform at most 2 operations. | ||
|
||
**Constraints:** | ||
|
||
* `2 <= nums.length <= 2000` | ||
* `1 <= nums[i] <= 1000` |
34 changes: 34 additions & 0 deletions
34
...kotlin/g3001_3100/s3047_find_the_largest_area_of_square_inside_two_rectangles/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
package g3001_3100.s3047_find_the_largest_area_of_square_inside_two_rectangles | ||
|
||
// #Medium #Array #Math #Geometry #2024_03_06_Time_753_ms_(40.42%)_Space_57.7_MB_(72.34%) | ||
|
||
import kotlin.math.max | ||
import kotlin.math.min | ||
import kotlin.math.pow | ||
|
||
class Solution { | ||
fun largestSquareArea(bottomLeft: Array<IntArray>, topRight: Array<IntArray>): Long { | ||
val n = bottomLeft.size | ||
var maxArea: Long = 0 | ||
for (i in 0 until n) { | ||
val ax = bottomLeft[i][0] | ||
val ay = bottomLeft[i][1] | ||
val bx = topRight[i][0] | ||
val by = topRight[i][1] | ||
for (j in i + 1 until n) { | ||
val cx = bottomLeft[j][0] | ||
val cy = bottomLeft[j][1] | ||
val dx = topRight[j][0] | ||
val dy = topRight[j][1] | ||
val x1 = max(ax, cx) | ||
val y1 = max(ay, cy) | ||
val x2 = min(bx, dx) | ||
val y2 = min(by, dy) | ||
val minSide = min((x2 - x1), (y2 - y1)) | ||
val area = max(minSide.toDouble(), 0.0).pow(2.0).toLong() | ||
maxArea = max(maxArea, area) | ||
} | ||
} | ||
return maxArea | ||
} | ||
} |
53 changes: 53 additions & 0 deletions
53
...3001_3100/s3047_find_the_largest_area_of_square_inside_two_rectangles/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
3047\. Find the Largest Area of Square Inside Two Rectangles | ||
|
||
Medium | ||
|
||
There exist `n` rectangles in a 2D plane. You are given two **0-indexed** 2D integer arrays `bottomLeft` and `topRight`, both of size `n x 2`, where `bottomLeft[i]` and `topRight[i]` represent the **bottom-left** and **top-right** coordinates of the <code>i<sup>th</sup></code> rectangle respectively. | ||
|
||
You can select a region formed from the **intersection** of two of the given rectangles. You need to find the **largest** area of a **square** that can fit **inside** this region if you select the region optimally. | ||
|
||
Return _the **largest** possible area of a square, or_ `0` _if there **do not** exist any intersecting regions between the rectangles_. | ||
|
||
**Example 1:** | ||
|
||
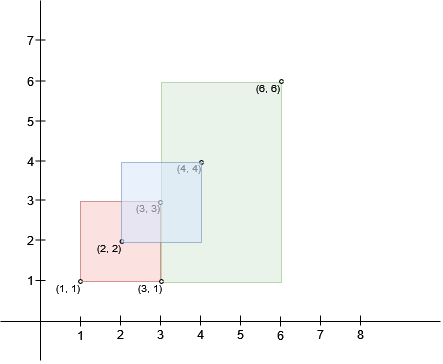 | ||
|
||
**Input:** bottomLeft = [[1,1],[2,2],[3,1]], topRight = [[3,3],[4,4],[6,6]] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** A square with side length 1 can fit inside either the intersecting region of rectangle 0 and rectangle 1, or the intersecting region of rectangle 1 and rectangle 2. Hence the largest area is side \* side which is 1 \* 1 == 1. | ||
|
||
It can be shown that a square with a greater side length can not fit inside any intersecting region. | ||
|
||
**Example 2:** | ||
|
||
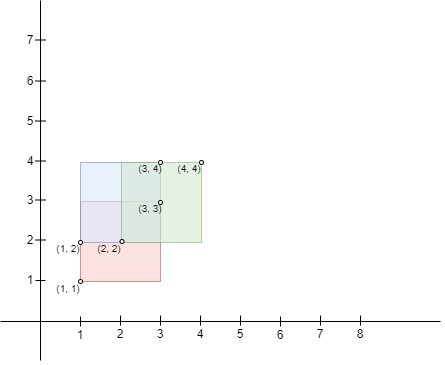 | ||
|
||
**Input:** bottomLeft = [[1,1],[2,2],[1,2]], topRight = [[3,3],[4,4],[3,4]] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** A square with side length 1 can fit inside either the intersecting region of rectangle 0 and rectangle 1, the intersecting region of rectangle 1 and rectangle 2, or the intersection region of all 3 rectangles. Hence the largest area is side \* side which is 1 \* 1 == 1. | ||
|
||
It can be shown that a square with a greater side length can not fit inside any intersecting region. Note that the region can be formed by the intersection of more than 2 rectangles. | ||
|
||
**Example 3:** | ||
|
||
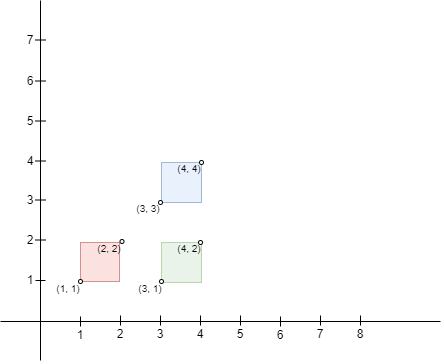 | ||
|
||
**Input:** bottomLeft = [[1,1],[3,3],[3,1]], topRight = [[2,2],[4,4],[4,2]] | ||
|
||
**Output:** 0 | ||
|
||
**Explanation:** No pair of rectangles intersect, hence, we return 0. | ||
|
||
**Constraints:** | ||
|
||
* `n == bottomLeft.length == topRight.length` | ||
* <code>2 <= n <= 10<sup>3</sup></code> | ||
* `bottomLeft[i].length == topRight[i].length == 2` | ||
* <code>1 <= bottomLeft[i][0], bottomLeft[i][1] <= 10<sup>7</sup></code> | ||
* <code>1 <= topRight[i][0], topRight[i][1] <= 10<sup>7</sup></code> | ||
* `bottomLeft[i][0] < topRight[i][0]` | ||
* `bottomLeft[i][1] < topRight[i][1]` |
50 changes: 50 additions & 0 deletions
50
src/main/kotlin/g3001_3100/s3048_earliest_second_to_mark_indices_i/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,50 @@ | ||
package g3001_3100.s3048_earliest_second_to_mark_indices_i | ||
|
||
// #Medium #Array #Binary_Search #2024_03_06_Time_223_ms_(75.00%)_Space_44.7_MB_(33.33%) | ||
|
||
class Solution { | ||
fun earliestSecondToMarkIndices(nums: IntArray, changeIndices: IntArray): Int { | ||
val n = nums.size | ||
if (nums.isEmpty() || changeIndices.isEmpty()) { | ||
return 0 | ||
} | ||
val last = IntArray(n) | ||
last.fill(-1) | ||
for (i in changeIndices.indices) { | ||
changeIndices[i] -= 1 | ||
} | ||
var low = 0 | ||
var high = changeIndices.size - 1 | ||
while (low < high) { | ||
val mid = low + (high - low) / 2 | ||
if (isPossible(mid, nums, changeIndices, last)) { | ||
high = mid | ||
} else { | ||
low = mid + 1 | ||
} | ||
} | ||
return if (isPossible(low, nums, changeIndices, last)) low + 1 else -1 | ||
} | ||
|
||
private fun isPossible(s: Int, nums: IntArray, changeIndices: IntArray, last: IntArray): Boolean { | ||
val n = nums.size | ||
last.fill(-1) | ||
for (i in 0..s) { | ||
last[changeIndices[i]] = i | ||
} | ||
var marked = 0 | ||
var operations = 0 | ||
for (i in 0..s) { | ||
if (i == last[changeIndices[i]]) { | ||
if (nums[changeIndices[i]] > operations) { | ||
return false | ||
} | ||
operations -= nums[changeIndices[i]] | ||
marked++ | ||
} else { | ||
operations++ | ||
} | ||
} | ||
return marked == n | ||
} | ||
} |
Oops, something went wrong.